Add Logging to a Net Core Console App
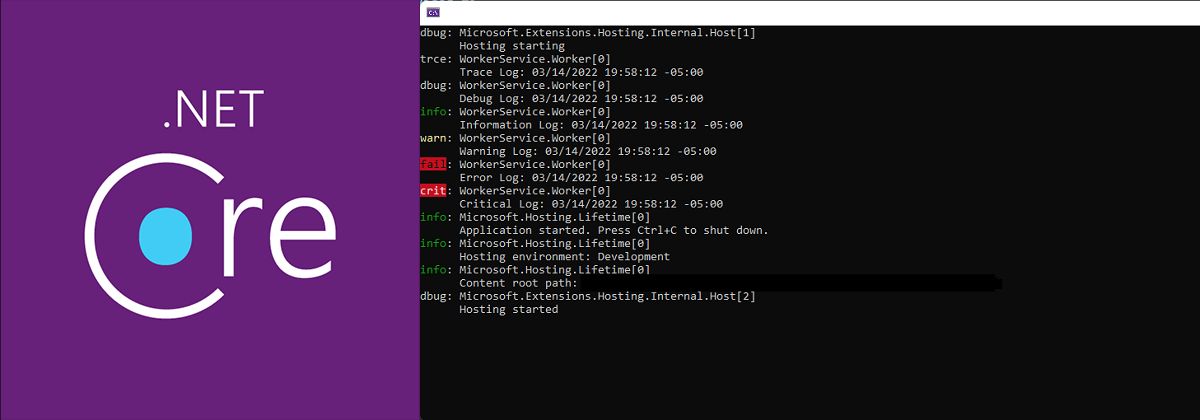
Logging is an essential feature of every application; it allows us to find and fix errors, handle warnings, and record information while debugging. In this article, we will check what does .NET Core gives us out of the box and how we can add more logging capabilities using third-party packages.
We will take as the starting point the console application from the previous post; it was created using the Worker Service template that is part of the .NET SDK. We will get the code that follows out of the box:
By default, the Host.CreateDefaultBuilder() call will add the following log providers to our console application:
- Console: Logs output to the console, logs are not saved.
- Debug: Logs to the debug output window.
- EventSource: Sends log output to a cross-platform event source. The dotnet-trace tool can be used to collect information from this provider.
- EventLog: sends log output to the Windows Event Log. These entries can be Windows Event Viewer.
Microsoft supports logging levels that will allow us to configure the logs' verbosity.
The higher the level, the fewer log records we will see.
The log levels available are the following:
Trace(0) < Debug(1) < Information(2) < Warning(3) < Error(4) < Critical(5) < None(6)
By default, the log level in the appsettings.json and appsettings.development.json will be "Information". Let's do a little experiment; let's change the log level to Trace for "Default" and None for "Microsoft.Hosting.Lifetime". Now we will add some lines to record every log level we ha available.
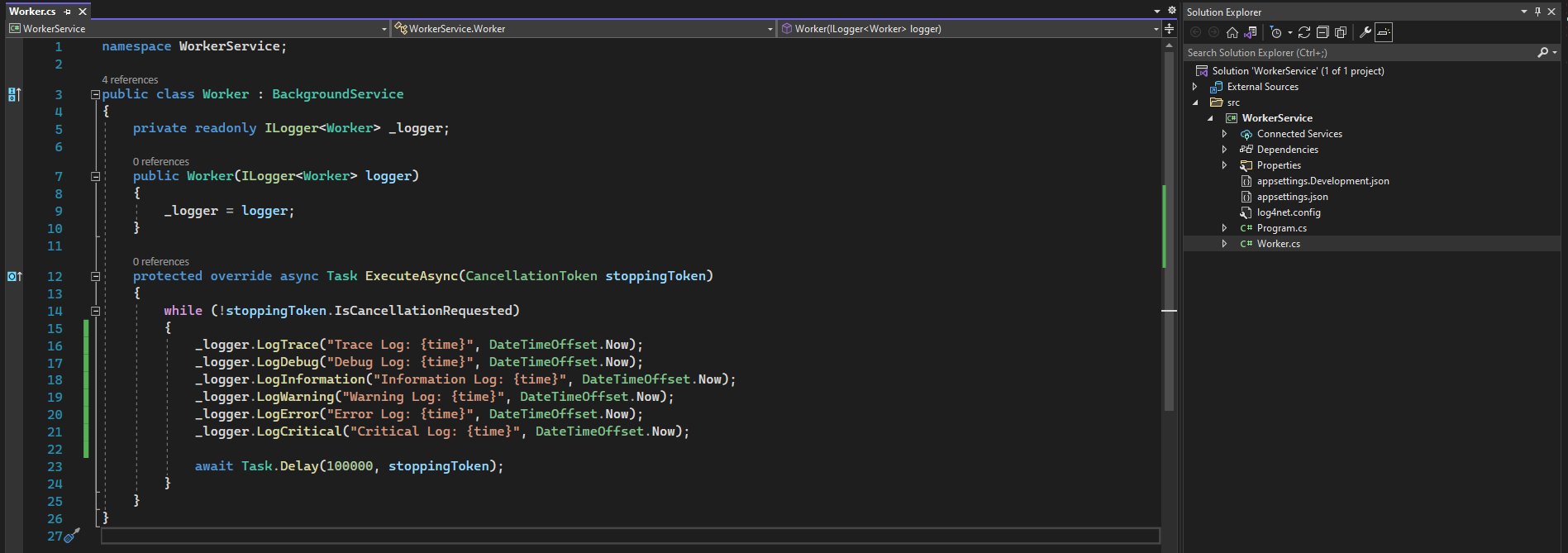
We should see results like this on the console:
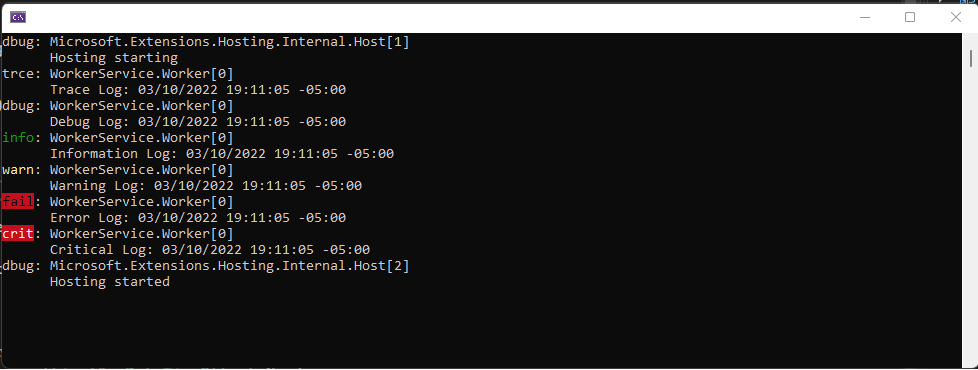
And warnings and errors in the Windows Event Viewer, this is because the default logging level for this provider is Warning.
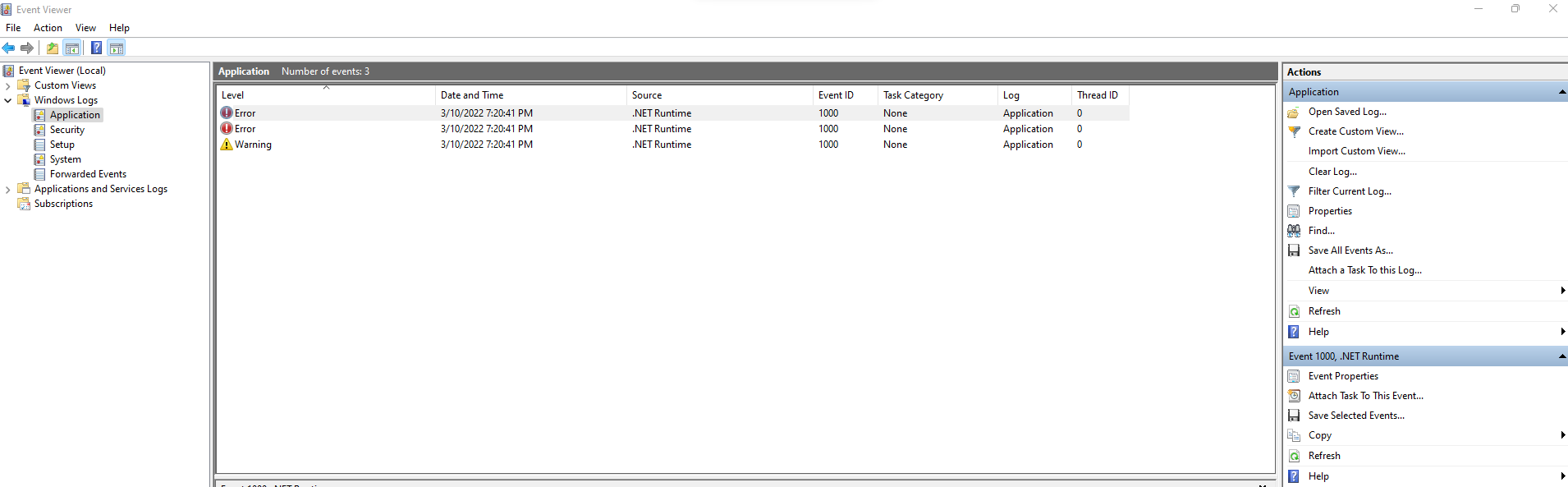
The Event Viewer will display two errors because we logged one of them as Error and the other one as Critical.
As you may have alredy notice, Microsoft does not include a logging provider for files, you will have to use a third-party to accomplish it. There are several options nowadays, some of them are: Log4Net, Serilog, NLog, and Sentry. We will configure and inspect a couple of them.
Adding Log4Net
Install Log4Net
Installs last version of Log4Net package
dotnet add src/WorkerService package log4net --version 2.0.14
Install Log4Net extensions
Install Log4Net extensions, this is a package created by the community outside of the official Log4Net packages that will allow us to wire up logging to the Host object and provide a bridge between Microsoft logging and Log4Net.
dotnet add src/WorkerService package microsoft.extensions.logging.log4net.aspnetcore
You will need a couple of modifications along the packages that we have just added. Basically we need to wire up Log4Net in the program class, and we need a configuration file to set our log appenders.
The logging leves supported by Log4Net are the following: ALL > INFO > WARN > ERROR > FATAL > OFF.
The extensions package will take care of translating the levels provided by Microsoft to the levels available
in Log4Net.
The advantage of using the Log4Net extensions is that we can capture all the built-int NET Core messages such as: "Hosting Starting", "Hosting Started", "Host Stoppping", "Host stopped". The reason of this is that NET Core will only write its internal messages to the ILoggerFactory instance that is created when the application starts.