Angular CLI Cheat sheet [#7]
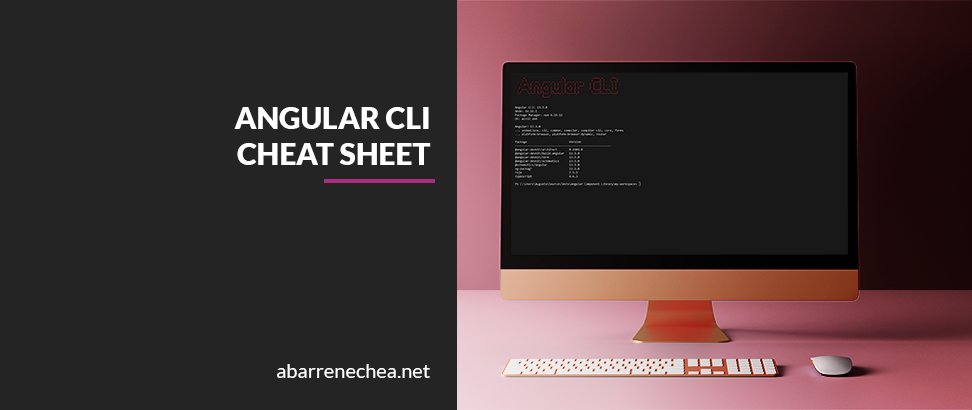
The Angular CLI is a tool from the Angular team that helps with code generation and follows best practices, in this post I have included some of the commands I use in my day to day at work. If you are not using the CLI now you are probably working on manual tasks that could be automated, for example when it comes to creating a new component the CLI provides a command that creates four files, each of them with base code. These are:
- componentname.ts: the component class in a typescript file
- componentname.html: the html template for the new component
- componentname.css: the styles file for the new component
- componentname.spec.ts: the unit test file with base code
With the CLI you can autogenerate code for components, services, run unit tests, serve your application, format and lint your code. You will find a lot of useful commands in the Angular CLI docummentation page: https://cli.angular.io/
Keep in mind that most of these commands have options and many options also have an alias,
for example to build your application and start a server you can run
ng serve --open
or ng serve -o
Remember that you can always ask for help to the CLI using the help command, for example try: ng help
Framework installation and version
Install Angular CLI
Install the Angular CLI, -g option indicates that it will be installed globally.
npm install -g @angular/cli
Uninstall Angular CLI
If you have a different version than the one you need you may want to remove your current Angular CLI before installing the new one.
npm uninstall -g @angular/cli
Check current Angular version
Verify what Angular, Angular CLI, Node and some other Angular components version.
ng version
ng v
Create an Angular Application
Create an empty workspace
If you are thinking about having multiple applications or libraries you can create an empty workspace where you can add projects and libraries as needed.
ng new my-workspace --create-application=false
Generate Angular building blocks
Create a library
Creates an Angular library
ng generate library [name] --prefix [prefix]
Create a module
Creates an Angular module
ng generate module [name]
ng generate module [name] --route [rout-path] --module [module-name]
Generate a component
Generates a new component including html, css and typescript files for component and tests.
ng generate c [componentname]
Module option indicates the declaring Angular module.
ng generate c [path]/[component-name] --module [module-name]
The flat option will indicate that a folder will not be created.
ng generate c [componentname] -flat
Run through without making changes, this will allow you verify what files and where would they be created.
ng generate c [componentname] --dry-run
Generate a Service class
Generates a service class and a file with a template for the unit tests.
ng generate service [ServiceName]
Generates a Pipe class
Generates a pipe class and a file with a template for unit tests.
ng generate pipe [PipeName]
Development Cycle
Serve application
Spin a server and serve the web page
Full command
ng serve --open
ng serve -o
Spin a server and serves the web page, this command will actually run whatever is set in your package.json file. By default an Angular project is created with an "ng serve" command for the npm start.
npm start
Linting
This command will use TSLint to check your code and look for style or programmatic errors.
ng lint
Run tests
ng test
Run tests and generate code coverage report. Full command
ng test --code-coverage
Run tests and generate code coverage report
ng test -cc